Tutorial: E-field modeling using simNIBS
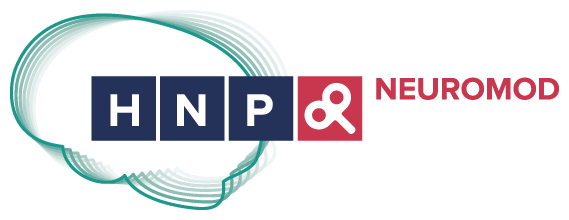
SimNIBS is a free and open source software package for the Simulation of Non-invasive Brain Stimulation. It allows for realistic calculations of the electric field induced by transcranial magnetic stimulation (TMS) and transcranial electric stimulation (TES).
It is a powerful tool to:
1. generate head meshes based on structural MRI
2. estimate and visualize individualized electrical fields generated by tDCS or TMS
3. calculate the optimal tDCS / TMS parameters (position / amplitude) to target specific brain regions
You can download and read about simNIBS on the official website HERE.
Installation
- Download and install the latest version of simNIBS for Windows / Linux / Mac using the following link : https://simnibs.github.io/simnibs/build/html/installation/simnibs_installer.html
Generation of head meshes
- The necessary steps to obtain a high resolution individualized head mesh are detailed HERE.
- To create individualized models, SimNIBS requireas a T1-weighted image. T2-weighted images are optional, but highly recommended.
- Specific sequence parameters can be seen at Nielsen et al., NeuroImage, 2018.
- Headreco is the preferred method (and the fastest), but requires Matlab.
E-field modeling: TMS
- SimNIBS offers both Python and MATLAB interfaces for setting up and running simulations. In both, a set of nested structures is used to define the simulation, and then the command run_simnibs is used to run the simulation.
- You can find detailed explanation on the various commands on the official website HERE.
- Here we detail a Python script for TMS:
from simnibs import sim_struct, run_simnibs # Initalize a session s = sim_struct.SESSION() # Name of head mesh s.fnamehead = 'ernie.msh' # Output folder s.pathfem = 'tutorial/'
# Initialize a list of TMS simulations tmslist = s.add_tmslist() # Select coil tmslist.fnamecoil = 'Magstim_70mm_Fig8.nii.gz'
# Initialize a coil position pos = tmslist.add_position() # Select coil centre pos.centre = 'C1' # Select coil direction pos.pos_ydir = 'CP1'
# Add another position pos_superior = tmslist.add_position() # Centred at C1 pos_superior.centre = 'C1' # Pointing towards Cz pos_superior.pos_ydir = 'Cz' run_simnibs(s)
E-field modeling: tDCS
- SimNIBS offers both Python and MATLAB interfaces for setting up and running simulations. In both, a set of nested structures is used to define the simulation, and then the command run_simnibs is used to run the simulation.
- You can find detailed explanation on the various commands on the official website HERE.
- Here is detailed a typical tDCS script using Python:
from simnibs import sim_struct, run_simnibs # Initalize a session s = sim_struct.SESSION() # Name of head mesh s.fnamehead = 'ernie.msh' # Output folder s.pathfem = 'tutorial/'
# Initialize a tDCS simulation tdcslist = s.add_tdcslist() # Set currents tdcslist.currents = [-1e-3, 1e-3]
# Initialize the cathode cathode = tdcslist.add_electrode() # Connect electrode to first channel (-1e-3 mA, cathode) cathode.channelnr = 1 # Electrode dimension cathode.dimensions = [50, 70] # Rectangular shape cathode.shape = 'rect' # 5mm thickness cathode.thickness = 5 # Electrode Position cathode.centre = 'C3' # Electrode direction cathode.pos_ydir = 'Cz'
# Add another electrode anode = tdcslist.add_electrode() # Assign it to the second channel anode.channelnr = 2 # Electrode diameter anode.dimensions = [30, 30] # Electrode shape anode.shape = 'ellipse' # 5mm thickness anode.thickness = 5 # Electrode position anode.centre = 'C4'
run_simnibs(s)
tDCS optimization based on target
- When using this feature in a publication, please cite Saturnino, G. B., Siebner, H. R., Thielscher, A., & Madsen, K. H. (2019). Accessibility of cortical regions to focal TES: Dependence on spatial position, safety, and practical constraints. NeuroImage, 203, 116183.
- Step 1: Create a leadfield based on electrode location
from simnibs import sim_struct, run_simnibs
tdcs_lf = sim_struct.TDCSLEADFIELD()
# head mesh
tdcs_lf.fnamehead = ‘ernie.msh’
# output directory
tdcs_lf.pathfem = ‘folder_leadfield’
tdcs_lf.eeg_cap=’electrodes_location.csv’
# run the simulations
run_simnibs(tdcs_lf)
- Step 2: optimize electrode location and amplitude based on leadfield.
import simnibs
# Initialize structure
opt = simnibs.opt_struct.TDCSoptimize()
# Select the leadfield file
opt.leadfield_hdf = ‘folder_leadfield/ernie_leadfield_electrodes_location.hdf5’
# Select a name for the optimization
opt.name = ‘optimization/dual_target’
# Select a maximum total current (in A)
opt.max_total_current = 2e-3
# Select a maximum current at each electrodes (in A)
opt.max_individual_current = 1e-3
# Select a maximum number of active electrodes (optional)
opt.max_active_electrodes = 6
# Define optimization target
target1 = opt.add_target()
# Position of target, in subject space!
# please see tdcs_optimize_mni.py for how to use MNI coordinates
target1.positions = [-12, 87, 48]
# Intensity of the electric field (in V/m)
target1.intensity = 0.2
target2 = opt.add_target()
target2.positions = [-81, -54, 16]
target2.intensity = 0.2 # negative value revert the direction
# Run optimization
simnibs.run_simnibs(opt)
- You can find more details on the official website HERE.
TMS optimization based on target
- SimNIBS can help finding the best TMS position for stimulating a certain target. This is done by searching coil positions in a grid around the target position and turning the coil at various angles.
- When using this feature in a publication, please cite Weise, K., Numssen, O., Thielscher, A., Hartwigsen, G., & Knösche, T. R. (2020). A novel approach to localize cortical TMS effects. Neuroimage, 209, 116486.
- Typical script:
from simnibs import opt_struct # Initialize structure tms_opt = opt_struct.TMSoptimize() # Select the head mesh tms_opt.fnamehead = 'ernie.msh' # Select output folder tms_opt.pathfem = 'tms_optimization/' # Select the coil model tms_opt.fnamecoil = 'Magstim_70mm_Fig8.nii.gz' # Select a target for the optimization tms_opt.target = [-43.4, -20.7, 83.4] # Run optimization to get optimal coil position opt_pos=tms_opt.run()
- You can find more details on the official website HERE.